Let's start with a simple example of testing authentication scenarios using Playwright and TypeScript. We'll focus on these three core scenarios:
Prerequisites
- Node.js installed
- Playwright installed
- VS Code with Playwright extension
Login
Let's start with the login scenario. We'll do it manually first to understand the steps and then automate it later. Go to this site Practice E-commerce, and perform the following scenarios manually. Then find the elements and automate them using Playwright.
Login Scenarios
Positive Scenario:
- Provide valid credentials (username/email and password). Then, verify successful login by checking for the presence of a specific element on the home page (e.g., user profile, dashboard).
Negative Scenario:
- Provide invalid credentials (incorrect username/email or password). Then, verify that an error message is displayed (e.g., "Invalid credentials").
- Test for empty fields (username/email or password).
Write a test
Create a new test file login.spec.ts
in the tests
folder and add the following code for the Positive Login scenario.
import test from "@playwright/test"
test('Should login successfully with valid credentials', async ({ page }) => {
// Go to Codemo.dev - Practice E-commerce site
await page.goto('https://codemo.dev/practice/shop/products')
})
First, we need to:
- Import
test
from Playwright - Add a test method (test case) with the title
- Add
page
as a Playwright built-in fixture that provides a browser context to interact with the web page, such as navigating, finding elements, and interacting with them - Add a step to navigate to the site using the
goto
method. This method needs async/await keywords to handle the asynchronous operation (JavaScript promise) because many Playwright methods return a promise. We can hover over thegoto
method to see the return type isPromise<null | Response>
.
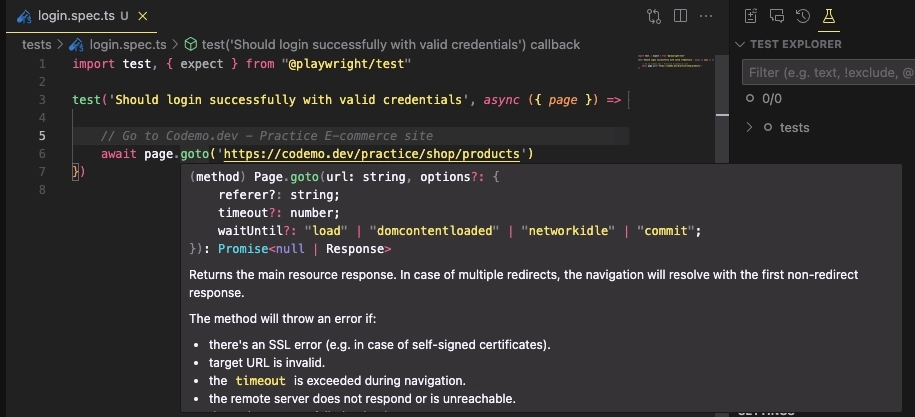
Then, write the rest to find and fill the username and password fields, click the login button, and verify the successful login.
import test, { expect } from "@playwright/test"
test('Should login successfully with valid credentials', async ({ page }) => {
// Go to Codemo.dev - Practice E-commerce site
await page.goto('https://codemo.dev/practice/shop/products')
// Find Username input field and fill it with 'admin'
await page.getByLabel('Username').fill('admin')
// Find Password input field and fill it with 'wowTesting123!'
await page.getByLabel('Password').fill('wowTesting123!')
// Expects the Login button to be enabled
await expect(page.getByRole('button', { name: 'Login' })).toBeEnabled()
// Click on the Login button
await page.getByRole('button', { name: 'Login' }).click()
// Expects the Admin button to be visible
await expect(page.getByRole('button', { name: 'Admin' })).toBeVisible()
})
Run the test
To run the test, execute the following command in the terminal:
// Run the specific test file
npx playwright test tests/login.spec.ts
// or run the specific test case
npx playwright test -g "Should login successfully with valid credentials"
Or use the Playwright Test extension to run the test directly from the editor by clicking on the ▶️ button next to the test case or test file.
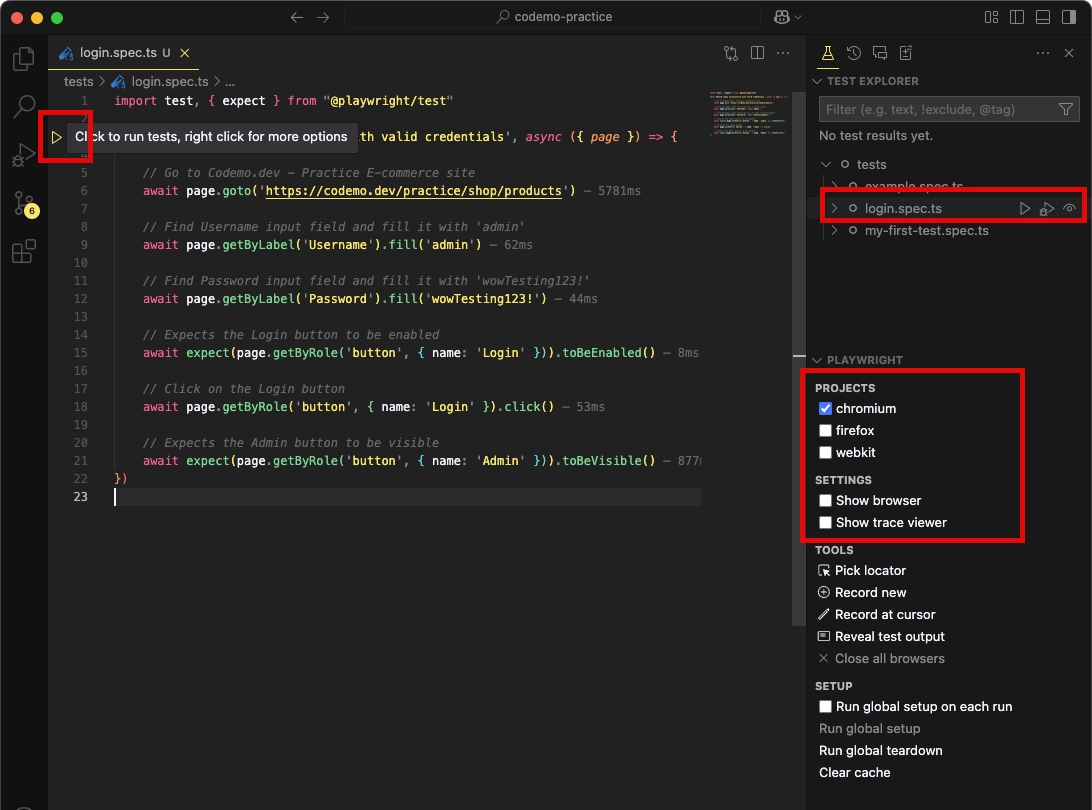
View the report
After running the test, we can see the test report in the terminal and/or show it in the browser. The report will show the test status, duration, and any errors or failures.
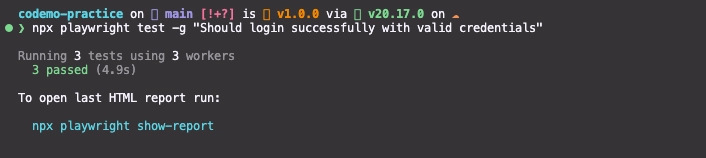
Run the following command to view the report:
npx playwright show-report
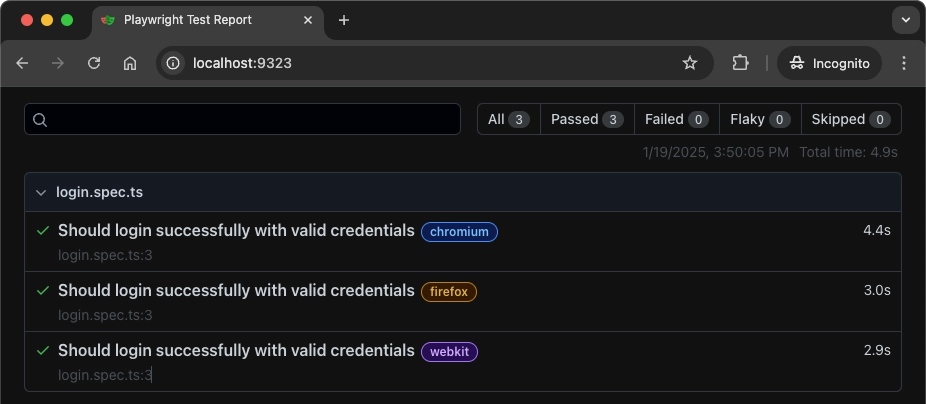
You can do it! Let's continue with the Login - Negative Scenarios 💪🏼. The source code is available on Github
Registration
The registration scenario is similar to the login scenario. We need to find the registration form elements, fill in the required fields, and click the register button. Then, we can verify the successful registration by checking for a specific element on the page.
import test, { expect } from "@playwright/test"
test('Should register successfully with valid information', async ({ page }) => {
// Go to Codemo.dev - Practice E-commerce site
await page.goto('https://codemo.dev/practice/shop/products')
// Click Create an account link
await page.getByRole('link', { name: 'Create an account' }).click()
// Input necessary information
await page.getByLabel('Username').fill('user_001')
await page.getByLabel('Email').fill('user_001@example.com')
await page.getByLabel('Password').fill('ThisisPassword1')
await page.getByText('Automation').click()
//Verify Register button is enabled then click
await expect(page.getByLabel('Register')).toBeEnabled()
await page.getByLabel('Register').click()
//Verify Register successful message is visible
await expect(page.getByText('Register successful')).toBeVisible()
})
Forgot Password
I will leave this scenario for you to practice. You can follow the same steps as the login and registration scenarios. I have set up an abnormal behavior case as a hidden bug for you to find 🐞 🚀
References
- Source code: Github