What are HTML Elements
HTML elements are the building blocks of an HTML page. An HTML element is written with a start tag <button>
, some content Click me
, and an end tag </button>
<body>
<p>This is a paragraph element</p> // p tag
<button type="button" class="btn-click">Click Me</button> // button tag
</body>
There are many types of tags available in HTML. Some of the common tags are: button
, input
, div
, h1
, select
, option
, label
, link
, etc.
We can find elements by using the browser developer tools. Right-click on the element and select Inspect
or Inspect Element
to open the developer tools.
How Playwright finds and interacts with HTML Elements
Find elements using Locators
Playwright provides a set of built-in locators to find elements on the page, such as:
page.getByRole()
page.getByText()
page.getByLabel()
page.getByPlaceholder()
page.getByAltText()
page.getByTitle()
These methods are auto-waiting and retry-ability, which means Locators represent a way to find elements on the page at any moment.
We can find almost all types of elements using these methods, but we can also use the page.locator()
method to find elements using CSS selectors or XPath (not recommended by Playwright).
Here is an example to find the Click Me
button:
<body>
<p>This is a paragraph element</p>
<button type="button" class="btn-click">Click Me</button>
</body>
We can find the button using the getByRole
method, where the role is button
and the name of the button is Click Me
page.getByRole('button', { name: 'Click Me' })
OR find the button using the locator
method
page.locator('button.btn-click')
page.locator('.btn-click')
Find element using Pick locator tool in VS Code
We can easily find elements using the Pick locator
tool in VS Code by adding the Playwright Test for VS Code extension (see this post for more details).
By clicking on the Pick locator
option, VS Code will open the browser, and we can select the element to get the locator.
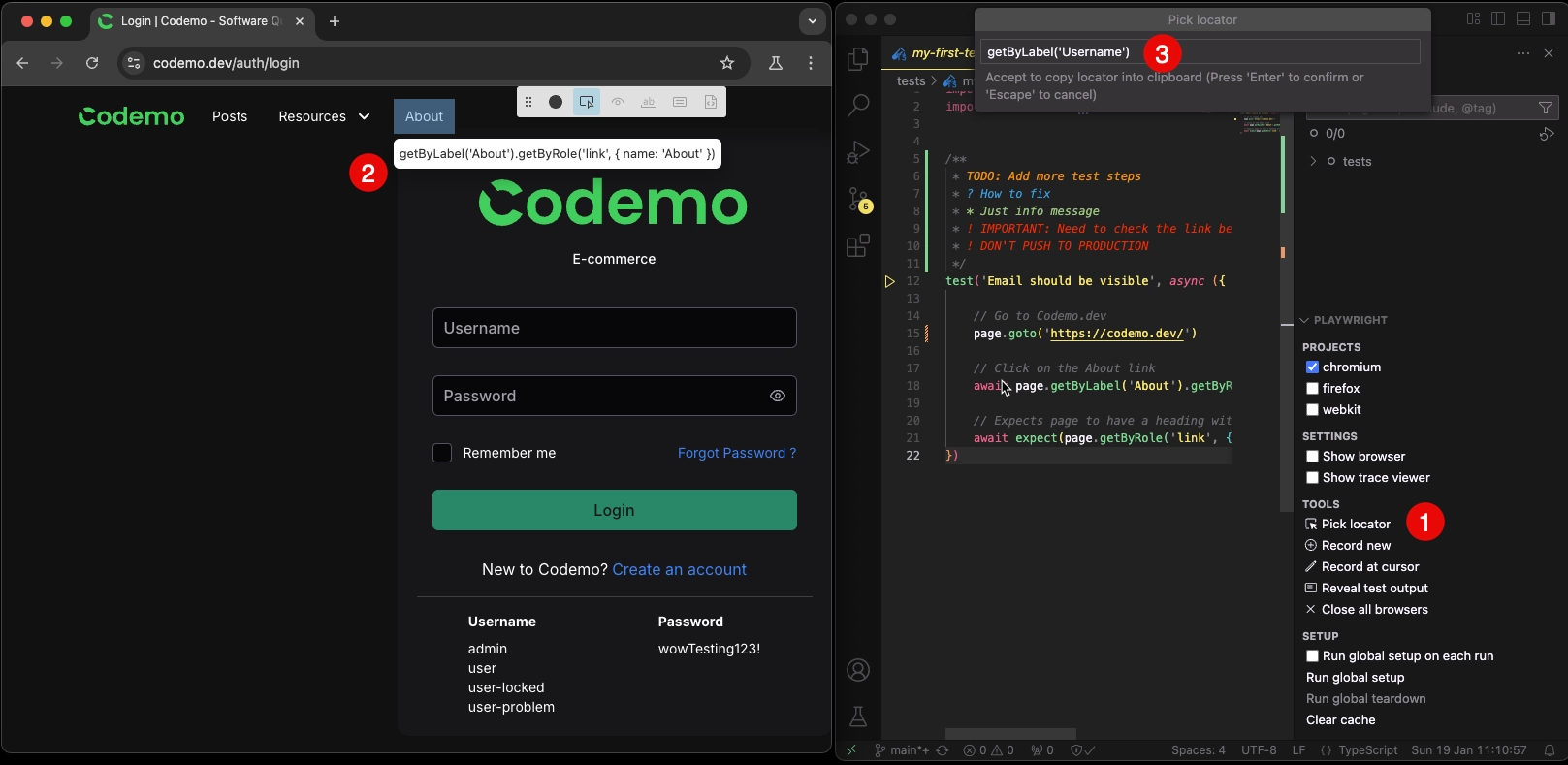
Interact with elements using Actions
In the previous example, we found the button element. Now we can interact with the button by clicking on it using the click()
method.
<body>
<p>This is a paragraph element</p>
<button type="button" class="btn-click">Click Me</button>
</body>
await page.getByRole('button', { name: 'Click Me' }).click()
Playwright provides a set of actions to interact with elements, such as click()
, fill()
, selectOption()
, check()
, press()
, etc.
Assert element state
We can assert the state of the element using the expect
method from the Playwright Assertions. For example, we can assert the button is disabled
or enabled
.
<body>
<p>This is a paragraph element</p>
<button type="button" class="btn-click" disabled>Click Me</button>
</body>
const buttonLocator = page.getByRole('button', { name: 'Click Me' })
await expect(buttonLocator).toBeDisabled() // ✅ The button is disabled, so the test will PASSED
await expect(buttonLocator).toBeEnabled() // ❌ This will FAILED
Challenges 💪🏼
You can practice to get more familiar with Playwright Locators and Actions using this site Practice E-commerce.
Scenarios:
- Go to the site and perform action Create an account, Forgot password and Login
- Login with example user and search for
Apple
item - Add
Apple
item to the cart and remove from the cart - Add
Apple
item to the cart and update the quantity - Checkout
This post covers the basics of finding and interacting with HTML elements using Playwright Locators and Actions. If you have any questions or feedback, feel free to reach out to me on LinkedIn or email me at codemo.dev@gmail.com
Happy Testing! 🚀